Programming Contest Guides
- Foreword
- Important Notice on Using PC2--Programming Contest Control System
- How to Select Winners
- Programming Practice for the Contest
- Programming Example(s)--how to put your code in a SINGLE Java file
- Program-example-1: all the code resides in the Java's main method:
- Program-example-2: creating/calling Java methods in a Single Java file:
- Program-example-3: using more than one Java class in a SingleJava file:
Foreword
-
The programming language will be Java.
-
You may NOT use any electronic forms of reference including the Internet.
- The only electronic document you can use is Java specifications/documents from the department's R:\Yi\SSU_ProgContest.
-
You may use any PAPER materials as you like, such as books, printouts of sample code.
-
Your submissions will be AUTOMATICALLY graded by the computer.
Important Notice on Using PC2--Programming Contest Control System
We
will use PC2 (Programming Contest Control System) to
automatically grade your submissions in the programming contest. We
will have training sessions before the contest and during the weeklly
meeting hours of Programming Club. Pay particular attention in
the following:
- We use standard input/output streams for
providing the inputs and outputs in testing your program.. You may not
use any other streams/sources for the inputs/inputs. That is, all the
inputs are from keyboard and all the outputs should be displayed on the
terminal.
- You must use terminal commands "javac " and "java" to
compile and execute your program (You may use any Java IDE/editor to
write/compile your code. But before you submit you program, you must
test it in the terminal environemnt with the two commands, which means
that you may need to necessary edition, for example, deleting package
line(s) in your code).
- You must put all your code for one question into a single Java file.See the following examples on how to do it.
- For each question, there are specific requirements on the inputs/outputs which you must follow strictly.
- For
example, you are supposed to write a Java code to display text "Hello,
Beifang" (without the quotations). If your code produce any of the
following outputs (without quotations, explanations are marked with
--), your program will not pass through the PC2:
- "Hello, beifang" --lowercase letter "b" used
- "Hello, Befang"--two whitespaces before "B" instead of one
- "Hello, Beifang."--an eding period "."
- It is always a good idea that
at first write a short Java code to read the inputs and output the
inputs you just read to make sure that you have obtained the correct
inputs---this may save you huge amount of your precious time!!
How to Select Winners
The selection of winners is based on the number of questions
answered correctly (passed through the grading system). If more than one
person has correctly answered the same number of questions. the person
who used least amount time wins.
- How PC2 calculate the working time:
- There is an inner clock for every question and all the clock starts ticking once the contest gets started.
- The
amount of time for each question you have solved is from the starting
time and the time when you submitted your solution for that question and if your solution is correct, i.e., your submission passed through PC2.
- Your
working time will be the sum of the times for all your (successful)
submissions. That means that if the contest will last for 120 minutes,
your working time might be well above 120 minutes (for example, 500
minutes, if we have more than four questions and you submitted each one
later).
- Suggestion: when you completed and successfully tested your code for a certain question, submit it immediately without delaying.
- If
your solution is wrong or the execution of your code takes much
more time (even though the output is correct), you will get a
message starting with "No.....". At this situation, you may examine
your code, your algorithms used in the implementaition. If you have
fixed the problem, you may submit again (remember, since your original
submission was not accepted by PC2, there is NO submission time record
associated with your submission for this question).
- For a contest question, how long it will take for a "correct" solution program to run before producing correct results?
- In
most cases, it usually takes less than 1~20 seconds including very
complicated testing cases. If longer execution will be needed for a
special case, announcements will be made for that question.
- PC2 usually provides enough time (about 40~60 secounds) to test your code.
- PC2 will get back to you with one of the following judgements:
- "Yes"
- "No - Compilation Error"
- "No - Run-time Error"
- "No - Time - limit Exceeded"
- "No - Wrong Answer"
- "No...." (such as output format errro, .... and etc.)
- ("Yes" is the only judgement for a correct solution)
- You may get "No - Time - limit Exceeded" message
even though your outputs for all the test cases are correct but the
excution time is too long. In this case, you need to figure out a
faster algorithm in your implementation.
- Efficient algorithm
is the core for the programming contest questions, particularly for the
challenging questions. It happens that an efficient algorithm can make
the execution millions, billion times faster!
Programming Practice for the Contest
The
SSU Programming Club has set up regular, weekly meetings and these
meetings provide excellent opportunities to the programming contest
practices. You need to complete most of the CSC201J topics either
through self-studying or taking the course for you to solve most of the
programming contest questions. A guiding principle in succeeding in the
contest is how to effectively, efficiently, and quickly apply what you have learned in the programming skills (tricks), not that you have to have mastered as many (Java) programming topics as possible.
The
following Java concepts (classes, methods, ..) are very frequently used
in any programming contests in Java programming languages:
- System.out.printf()
- fields in class Integer
- methods in class Math
- abs()
- ceil()
- floor()
- max()
- min()
- PI (field)
- E (field)
- methods in class String
- charAt()
- compareTo()
- concat()
- contains()
- isEmpty()
- indexOf()
- split()
- substring()
- toCharArray()
- toLowerCase()
- toUppercase()
- methods of class Scanner:
- hasNext()
- nextInt(), nextDouble(), nextLine()
- how to declare, define and use one dimmensional and two dimmensional arrays
- System.nanoTime() is not used in the contest question, but you may use
this method in programming practice in how to evaluate your
implementation of different algorithms for a certain same question.
The
following (algorithm) topics sometimes appear in very challenging
programming contest questions (if there are challenging questions). No
current CSC courses at SSU cover all these topics. But the
implementations of them are 100% covered in CSC201J. The SSU
Programming Club has selected these topics for programming practices.
- DP--Dynamic Programming
- String matching
- recursion
- modulus operator
- conversion of numbers (values) between different number systems
- Bitwise and bit shift operators
- (Contact Programming Club for more examples...)
Programming Example(s)--how to put your code in a SINGLE Java file
We
now use an exmple to explain how to put your Java code in a Single Java
file when you need to have more than one Java class and/or Java method.
Question:
Write a Java program to calculate the perimeters and areas of a set of rectangles.
Input: The first line of inputs provides the number of rectangles (i.e., an integer N). This first line will be followed by N
lines of 2 double numbers separated with a whitespace (i.e., each line
consisting of 2 double numbers) for the length and width of a rectangle
(the first number as the length and the second one as the width).
Output: N
lines of outputs, each of which consisting of 2 floating-point numbers
in 2 decimal places for perimeter and area of a rectangle. The first
line of output corresonds to the first rectangle of the input; the
second line to the second rectangle....
Input/output example:
Input:
2
3.0 4.0
3.1 4.1234
Output:
14.00 12.00
14.45 12.78
Program-example-1: all the code resides in the Java's main method:
Pay attention to the follwoing Java code. All the code is inside the Java's main method. The code is available here.
Important: The output consists only the required output in the required format (i.e., on a single line, 2 double numbers in 2 decimal places, separated with a signle white-space).--see line 18 You may not place any other texts/numbers!
.
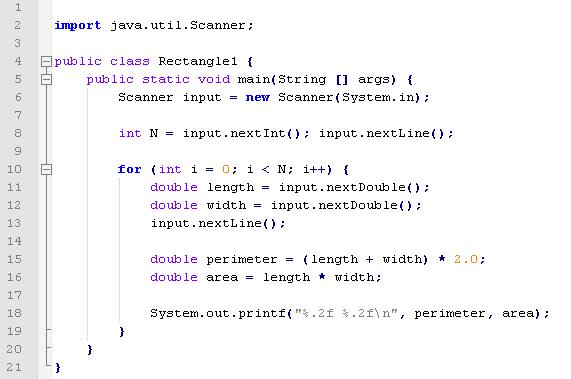
Program-example-2: creating/calling Java methods in a Single Java file:
In
the following Java code (for the same problem as the above), we create
two Java methods (i.e., recPerimeter() and recArea()) and call them in
the Java main method. The code is available here.
Important:
notice the brace at line 28 which means that the defined two methods
are within the same class. These two methods are declared as static.
Program-example-3: using more than one Java class in a SingleJava file:
In the following Java code (for the same problem as the above), we
create one more Java class, Rectangle, to solve the problem. The code is available here.
Important notice:
- The two classes are in the same Java file (whose name must be Rectangle3.java).
- The newly added class (i.e., Rectangle, lines from line 22 to line 36) is spearate from class Rectangle3 which contains main method (The brace on line 20 closes off class Rectangle3).
- Class Rectangle does not hav keyword public.